Add the following snippet to your HTML:
Let's have a look at how you could start measuring angles using the TLE5012Kit2Go Qualisys Mocap Machines
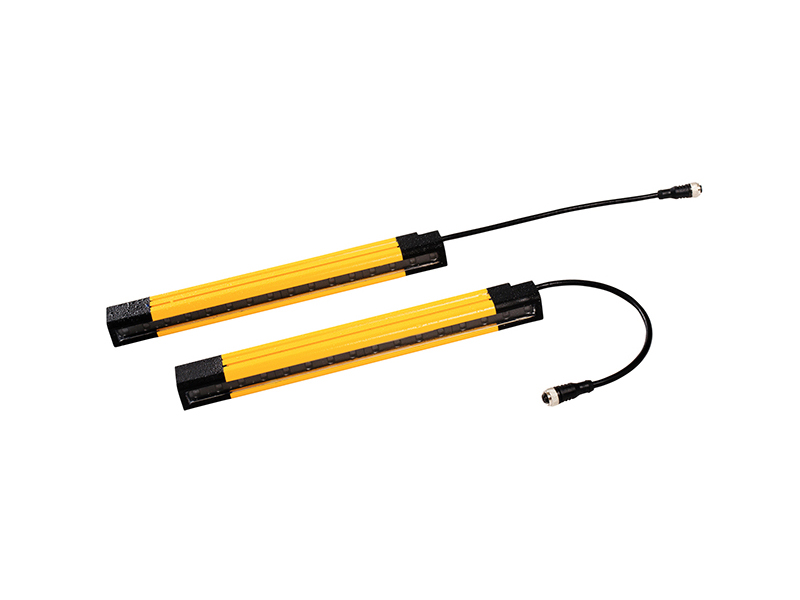
Read up about this project on
Let's have a look at how you could start measuring angles using the TLE5012Kit2Go
Greetings fellow makers! When thinking about any fancy project, you might almost certainly require some input. This input could potentially be a button, a microphone, a switch or even some fancy sensor like a radar. But what if there was something else. Maybe we could try to look at this from a different angle (*Badum TSSS*), AHA! What if you could use angle as input. As the title of this protip suggests, today we will be exploring using the TLE5012KIT2GO, which is an angle sensing solution packed in one PCB. (PSST it has a temperature sensor as well ;) )
The goal throughout this protip is that you:
The potential applications for an angle sensor in your project are limitless; it's like opening a new dimension for input. Take for example a project I worked on involving a critical gear rotation controlled by a motor, which was directly influenced by the angle sensor's readings.
But let's simplify things. Suppose you're prototyping, needing mechanical input for functionality testing. Traditional options like buttons or switches may spring to mind, providing straightforward binary input - 'on' or 'off', 'yes' or 'no'. But what if you need something more nuanced?
Enter the precision angle sensor, a device offering far more than a binary choice. It's like a 360-degree turntable of choices, transforming your project into a dynamic, interactive and detailed conversation. So let's explore the possibilities this tool offers.
Let's take a better look at the kit we've got over here:
This beautiful piece of circuitry consists of two main characters:
Of course as you could see in the photo there are other components involved, but all the other components are essentially working in the shadows for these two MVPs to operate correctly
There are four derivatives for the Sensor:
Note: If you just started your maker career you will most probably stick to SSC, and that's exactly what we will be using through out this protip!
For more concrete information about these protocols, check out the datasheet by clicking here!
And as we're at it, let's talk a bit about the specs:
Good Question! To answer it we need to understand how the sensor works.
The IC has these in-built components called Giant Magneto Resistance elements, or iGMR for short. These little guys are fantastic at measuring the 'sine' and 'cosine' components of the magnetic field. These might sound like scary math terms, but they're just ways of describing different aspects of the field, much like how we might describe a journey in terms of distance and direction.
Once the TLE5012B has these raw measurements (the sine and cosine), it gets to work processing them internally, just like solving a puzzle. By piecing together these parts, it's able to calculate the orientation, or angle, of the magnetic field.
So we just need a magnet and voila! As soon as the magnet is close enough to the sensor IC, it will detect it and calibrate itself to it's magnetic field. And if there is a change in the magnetic field lines getting to the IC (either the IC itself rotates or the magnet itself does) this rotation will be registered.
For a better representation we could take a look at some of the official accessories that Infineon provides. (These are also 3D printable and the files are on the website).
Before diving into programming the kit ourselves (which is extremely simple and straight to the point I promise!!!!) feel free to check out this presentation on the sensor and a graphical user interface tool that tests it and provides an extra interactive interface to see how the sensor works. (It's only three minutes)
Before we start using the board, we'll have to install its library, that makes our lives easier when programming.
ToInstall the library. In the Arduino IDE, go to the menu Sketch > Include library > Library Manager. Type TLE5012 and install the library.
Arduino code basically consists of two parts: a dynamic and a static part.
The static part or "the skeleton" as I like to call it: is just the part where all the initialization is done and we tell the Arduino essentially what it's connected to. The skeleton part is ran only once and then the Arduino jumps onto the dynamic part.
The dynamic part is the part, where we tell the Arduino "Hey I need you to do this and that, and in case of a certain scenario maybe do this" and so on. It's the part where we explicitly instruct the microcontroller to do stuff. This part is continuously ran, as long as there's power delivered to the microcontroller.
If you decided to not just copy the skeleton code and maybe dive into what constitutes the code then first of all I would like to congratulate you (truly,your boldness inspires me).
First, include the library at the top of your Arduino sketch:
Next, create an instance of the TLE5012Ino class:
This is like telling your Arduino "We have a TLE5012 sensor, and we're going to call it Tle5012Sensor.
Now we need to get in the setup function:
We also need to keep track of any errors that might occur when we're setting up or using the sensor. We'll do this with a variable called checkError:
At this point, checkError is set to NO_ERROR, because we haven't encountered any problems yet.
Now, we move on to the setup() function, which is where we'll initialize the sensor and set up communication with your computer.
We start the Serial communication:
The Serial.begin(9600); command starts the Serial communication at a baud rate of 9600. This will allow the Arduino to send data to your computer.
We want to make sure the Serial communication is set up correctly before we proceed, so we include this line:
Now, we're ready to start the TLE5012 sensor:
The Tle5012Sensor.begin(); command starts the sensor and returns an error code that gets stored in checkError.
We can then print this error code to the Serial monitor, which will help us diagnose any problems:
The Serial.println(checkError,HEX); command prints the error code in a hexadecimal format, which is a common format for error codes.
After another brief pause, we print a message to the Serial monitor to confirm that the initialization is done:
And that's it! You've set up your TLE5012 sensor with your Arduino. With this setup, your Arduino can check the sensor, let you know if there are any problems, and communicate the results to you over the Serial monitor.
The sensor provides a lot of bells and whistles to tinker with. The most important ones being the angle and temperature and here's the good news, using these functionalities is super simple (like really - it's just one line of code).
To get the angle all you have to do is call the getAngleValue() method. Additionally you have to feed this method with a variable (of type double), where it will store the angle values.
Let's say we want to create a variable, store the angle value in it and then print it out in the serial monitor. In that case all we'll do in the loop function is:
The angle value that is then returned is a value between -180 and 180 degrees.
Analogous to the angle values, you could also obtain the temperature reading with just a single method. All you have to use is the getTemperature() method. This method also takes in a variable of type double, where it saves the temperature values onto.
Let's say we want to create a variable and then store the temperature value in it and then print it out in the serial monitor. In that case all we'll have to do in the loop function is:
by just copy pasting we get the following code
when we run the following code in the Arduino IDE we get the following application
As you can see in the GIF above, the microcontroller constantly gives us new readings for the temperature and angle.
Now let's spice this up a little bit, let's try to see if we could use the angle sensor to mimic the input of a joystick. To do this all we'll have to do is use our previous code that we hooked up together. We will essentially just move our joystick addon up, down, left and right and just record the readings of the sensor. Based on that we will determine the value windows for each direction. After monitoring these values I just wrote if conditions so that in the dynamic part that's always running, the microcontroller read's the angle value from the sensor and then check's to see, to which direction does the angle value belong and so it prints that direction.
Additionally I used an integer value to represent to direction of input, so that I could use that further on if I decide to build an application, that is dependent on this input.
Let's see how the code looks like in action!
What extras does the library offer?
There are definitely more features that we did not talk about here, but feel free to explore what the library has to offer by checking out the Arduino code examples of the library. You could reach them by opening your Arduino IDE and hovering over File> Examples> TLE5012B
Angle Sensor vs 3D sensor?
2D Magnetic Sensors: These sensors measure magnetic fields in a flat plane (like the compass direction of a magnet relative to the sensor). They can't detect if a magnet is moving up or down.
3D Magnetic Sensors: These sensors measure magnetic fields in all three dimensions - not only can they detect the compass direction of a magnet, but they can also tell if it's moving up or down relative to the sensor. It's like having a full picture of the magnetic field's orientation and strength.
More Info about 3D Magnetic Sensors?
Interested in 3D magnetic Sensors? You're in luck because we also have a protip covering Infineon's 3D magnetic sensory solutions. (Click here for more details)
Angle Sensors (Magnetic 2D): These sensors use a magnetic field to determine the angular position or orientation of an object. They are non-contact devices, which means they don't need to physically touch the object whose position is being measured. This makes them more durable as they don't wear out from friction. They can also typically measure a full 360 degrees rotation and are less affected by environmental conditions like dust or dirt.
Potentiometers: These are a type of resistor with a variable resistance that can be manually adjusted. When used as angle sensors, they operate on the principle of mechanical rotation. As the potentiometer is turned, it changes the resistance, which can be measured and translated into an angle. However, they usually can't measure a full 360 degrees rotation, and being contact devices, they can wear out over time due to friction. Also, they are more affected by environmental conditions and require more maintenance.
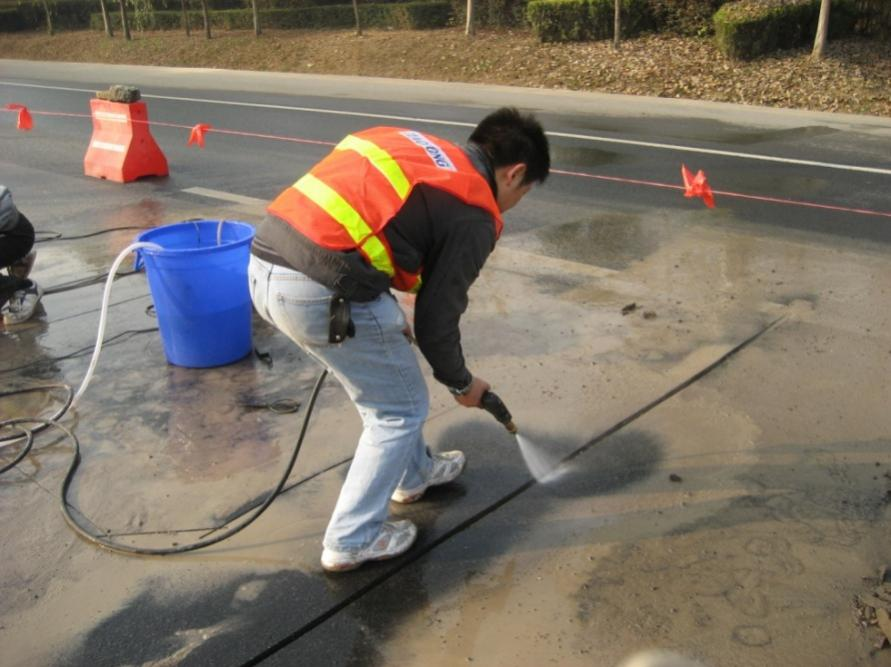
Wim Quartz Hackster.io, an Avnet Community © 2024